Axios를 이용하여 XML 데이터를 get하고 받은 데이터를 다시 JSON 포멧으로 변경하여 리스트로 출력하는 방법에 대해 포스트 하겠다.
Vue 프로젝트 생성 및 패키지 설치
먼저 예제에 사용할 신규 프로젝트 디렉토리를 생성한다. 그리고 필요한 패키지들을 npm 명령을 이용하여 설치한다.
vue create xml2json_test cd xml2json_test npm install axios xml-js
파싱하여 출력할 데이터 소스
참조 데이터는 영화진흥위원회에서 제공하는 Open API다. 아래 링크 데이터들은 박스오피스 데이터들인데 이를 받아서 JSON 포멧으로 변경한다.
소스 코드 수정
생성된 프로젝트 디렉토리에서 아래와 같이 파일을 수정한다.
src/main.js
import Vue from 'vue' import App from './App.vue' import axios from 'axios' Vue.config.productionTip = false Vue.prototype.$http = axios new Vue({ render: h => h(App), }).$mount('#app')
src/App.vue
App.vue 파일의 경우 기존에 작성되있던 코드를 모두 지우고 아래와 같이 변경한다.
<template> <div id="app"> <ul> <li v-for="item in items.boxOfficeResult.dailyBoxOfficeList.dailyBoxOffice" v-bind:key="item.movieNm"> {{ item.movieNm._text }} </li> </ul> </div> </template> <script> var convert = require('xml-js') export default { name: 'App', data () { return { items: [] } }, created () { this.$http.get('http://www.kobis.or.kr/kobisopenapi/webservice/rest/boxoffice/searchDailyBoxOfficeList.xml?key=430156241533f1d058c603178cc3ca0e&targetDt=20120101') .then((response) => { var xml = response.data var json = convert.xml2json(xml, { compact: true }) this.items = JSON.parse(json) }) } } </script>
출력 결과
수정을 끝낸 후 아래와 같이 작성된 내용을 서버에서 실행해본다.
vue 실행
npm run serve
서버 실행 후 http://localhost:8080 접속하면 아래와 같은 페이지를 확인할 수 있다.
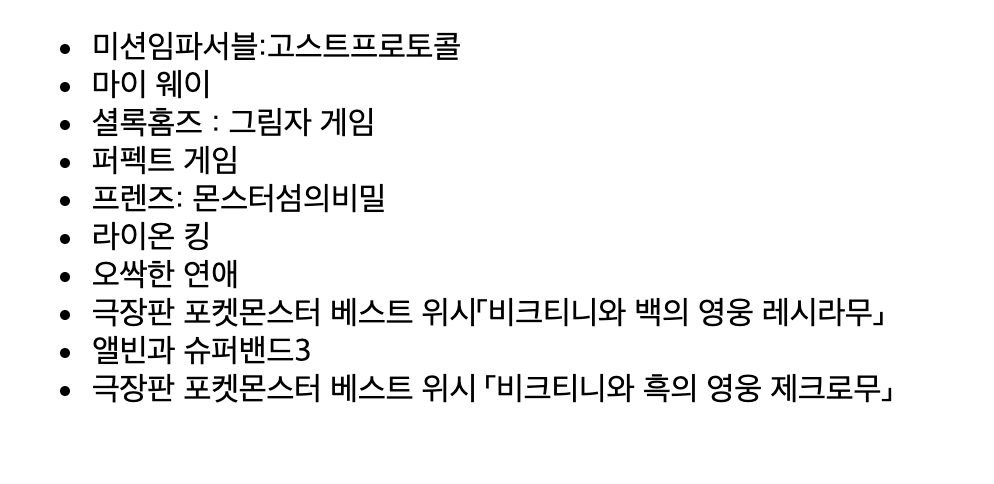